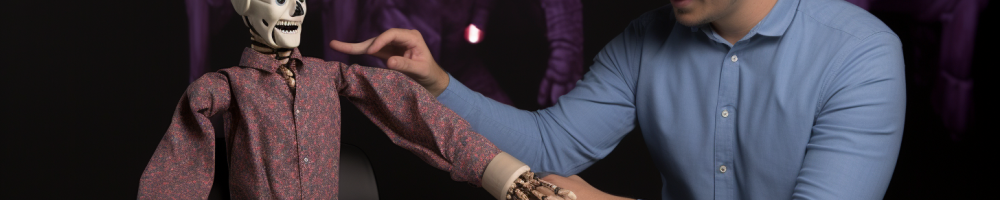
In this tutorial, we will explore the basics of using the headless browser Puppeteer.
What is Puppeteer?
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium through the DevTools protocol. It can be used for automating tasks in a browser, such as generating screenshots, crawling websites, and interacting with web pages programmatically.
Installation
To use Puppeteer, you first need to install it as a dependency in your project. You can do this by running the following command:
npm install puppeteer
Getting Started
Once Puppeteer is installed, you can require it and start using it in your JavaScript code. Here's a simple example that opens a new browser page and takes a screenshot:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://www.example.com');
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
Key Features
Puppeteer provides a range of powerful features that make it a versatile tool for browser automation:
- Full control over the browser: Puppeteer allows you to perform actions such as clicking, typing, and scrolling on web pages programmatically.
- Screenshot and PDF generation: You can take screenshots or generate PDFs of web pages using Puppeteer.
- Page automation: Puppeteer enables automatic form submission, navigation, and interaction with web pages.
- Network interception: Puppeteer can intercept and modify network requests, allowing you to simulate different network conditions.
- Headless execution: Puppeteer can run Chrome or Chromium in a headless mode, meaning there is no visible browser window.
- Crawling and scraping: Puppeteer can be used to crawl websites and scrape data from web pages.
Conclusion
Puppeteer is a powerful tool for automating tasks in a headless browser. Whether you need to generate screenshots, scrape data, or perform automated testing, Puppeteer provides a simple and convenient way to control Chrome or Chromium programmatically. With its wide range of features, Puppeteer is a great option for any JavaScript developer.