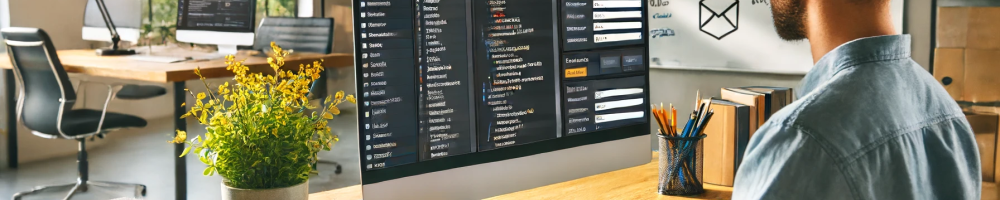
Sending emails programmatically is a common requirement for web applications. In Drupal 8, this can be efficiently managed using the core mail system. This article provides a step-by-step guide on how to send emails from your Drupal 8 modules.
Step 1: Implementing hook_mail()
Create a custom module, if you haven't one already. In your custom module, implement hook_mail()
to define the email templates. Here’s an example:
/**
* Implements hook_mail().
*/
function mymodule_mail($key, &$message, $params) {
$options = array(
'langcode' => $message['langcode'],
);
switch ($key) {
case 'my_mail':
$message['from'] = \Drupal::config('system.site')->get('mail');
$message['subject'] = t('Email Subject', [], $options);
$message['body'][] = t('Hello @name, this is a test email.', ['@name' => $params['name']], $options);
break;
}
}
Step 2: Sending the Mail
To send an email, you need to use the \Drupal::service()
to get the mail manager service and call the mail()
function. Below is an example for sending an email:
function mymodule_send_mail($to, $name) {
$mailManager = \Drupal::service('plugin.manager.mail');
$module = 'mymodule';
$key = 'my_mail';
$params['name'] = $name;
$langcode = \Drupal::currentUser()->getPreferredLangcode();
$send = true;
$result = $mailManager->mail($module, $key, $to, $langcode, $params, NULL, $send);
if ($result['result'] !== true) {
drupal_set_message(t('There was a problem sending your message and it was not sent.'), 'error');
}
else {
drupal_set_message(t('Your message has been sent.'));
}
}
Step 3: Testing and Debugging
Test your implementation by triggering the conditions that cause the email to send. Check the site's error logs and mail logs if you don’t receive the emails. Sometimes, emails sent by local environments don't go through because they get caught in spam filters or aren’t configured properly for sending outgoing mails.
Conclusion
Through these steps, you can set up and customize email sending in Drupal 8 according to your application's requirements. It crucially involves implementing hook_mail() hook and correctly configuring the mail manager.