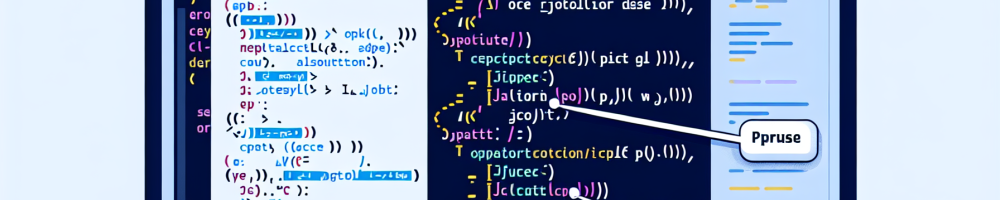
Web applications often require routing to handle different URLs correctly. In many web frameworks, you have the ability to pass arguments through routes to the callback functions that handle these routes. Sometimes, it's necessary to define these route parameters as optional to provide more flexible routing possibilities. This article will cover the basic method to define an optional argument in a route callback using common web frameworks.
Express.js Example
In Express.js, optional route parameters can be indicated by a question mark (?
) after the parameter name in the route definition. Here’s how you can do it:
const express = require('express');
const app = express();
app.get('/user/:name?', (req, res) => {
const name = req.params.name ? req.params.name : 'Guest';
res.send(`Hello, ${name}`);
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
Flask Example
In Flask, a Python web framework, optional parameters aren't directly supported in the route itself, but you can achieve similar functionality by providing multiple routes or using variable rules:
from flask import Flask, request
app = Flask(__name__)
@app.route('/user', defaults={'name': 'Guest'})
@app.route('/user/<name>')
def hello(name):
return f'Hello, {name}'
if __name__ == "__main__":
app.run()
In both cases, the key is to provide a mechanism where the parameter can default to a certain value if not provided, thereby making it optional.
Conclusion
Defining optional arguments in route callbacks allows developers to create more adaptive and comprehensive web applications. Depending on your chosen framework, the method to implement this feature can vary, but the underlying principle remains the same: provide functionality that safely handles cases where expected data might not always be provided.