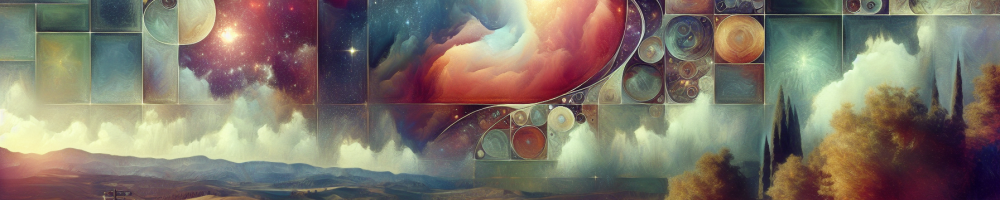
Puppeteer is a powerful Node library which provides a high-level API over the Chrome DevTools Protocol. It can be used for various browser automation tasks including, but not limited to, scraping content from websites, generating pre-rendered content from websites, and automating form submission.
Introduction to Puppeteer
Puppeteer effectively allows developers to programmatically control a Chrome (or Chromium) browser instance. It's widely used for testing web applications, taking screenshots of web pages, generating PDFs, and more.
Use Case: Removing Script Tags
One common use case in web scraping and automation with Puppeteer is the need to manipulate the HTML of the page, such as removing all script tags. This can be particularly useful for improving performance or ensuring that no tracking scripts are executed when loading the page programmatically.
Step-by-Step Guide to Remove Script Tags
- Set Up Puppeteer: Start by installing Puppeteer via npm (Node Package Manager).
- Launch the Browser: Write a script to launch a headless browser instance.
- Navigate to the Page: Load the webpage from which you want to remove script tags.
- Remove Script Tags: Use Puppeteer’s
evaluate
function to execute code in the context of the page. - Process or Save the Page: After removing the scripts, you can proceed with processing the page as needed. To save the resultant HTML to a file:
npm install puppeteer
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.evaluate(() => {
const scriptElements = document.querySelectorAll('script');
scriptElements.forEach(el => el.parentNode.removeChild(el));
});
const content = await page.content();
// fs (FileSystem) to write content to file
require('fs').writeFileSync('output.html', content);
await browser.close();
})();
Conclusion
By following these steps, you can use Puppeteer to remove script tags from any webpage. This method can enhance both the privacy and performance of automated web page manipulations. Keep in mind that any JavaScript-driven site features might not work correctly once you remove script tags, so use this approach judiciously.