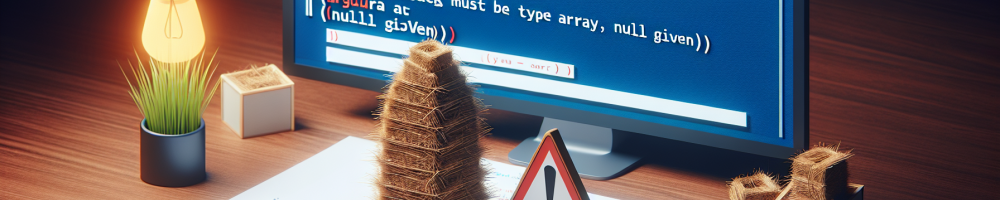
How to Resolve Laravel 9 Error: Argument 2 for 'in_array' must be of type array, null given
If you are encountering the error "Argument 2 for 'in_array' must be of type array, null given" in Laravel 9, don't worry. This is a common error that can be resolved easily by following a few steps.
Step 1: Check for Null Values
The error message indicates that the second argument passed to the 'in_array' function is null. This can happen if you are trying to check for the presence of a value in an array, but the array itself is null.
To resolve this error, you need to first check if the array is null before passing it to the 'in_array' function.
Step 2: Validate the Input
Another common cause of this error is passing invalid input to the 'in_array' function. Make sure that the array you are passing as the first argument is indeed an array and not null or any other data type.
You can use the 'is_array' function to validate the input before calling 'in_array' to avoid this error.
Step 3: Handle Null Values
If you expect the array to sometimes be null, you can add a check to handle this case gracefully. You can either initialize the array with an empty array if it is null or skip the 'in_array' check if the array is null.
Example Code
Here is an example code snippet that demonstrates how to handle null values when using the 'in_array' function in Laravel 9:
$array = $array ?? [];
if(is_array($array) && in_array($value, $array)) {
// Value is found in the array
} else {
// Value is not found in the array
}
By following these steps and making sure to validate the input before calling the 'in_array' function, you can easily resolve the error "Argument 2 for 'in_array' must be of type array, null given" in Laravel 9.