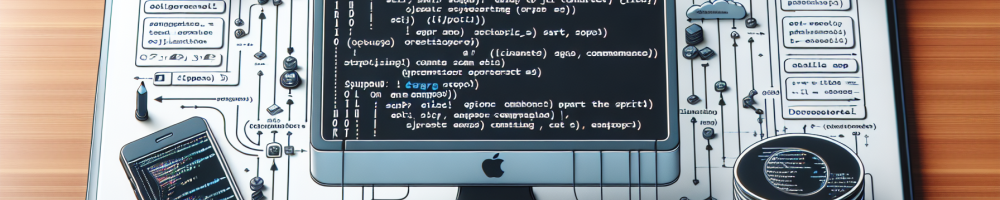
Implementing Error Handling in Specific Parts of a PostgreSQL Script
PostgreSQL is a powerful open-source relational database management system that is widely used for managing large volumes of data. When writing scripts in PostgreSQL, it is important to incorporate error handling to ensure that any potential issues are caught and handled appropriately. Implementing error handling in specific parts of a PostgreSQL script can help improve the overall robustness and reliability of the script.
1. Using TRY...CATCH Blocks
One common way to implement error handling in a PostgreSQL script is to use TRY...CATCH blocks. These blocks allow you to wrap specific parts of your script in a try block and catch any errors that may occur. For example:
BEGIN
-- Main script logic here
-- Error handling
BEGIN
-- Specific part of the script where errors may occur
EXCEPTION
WHEN others THEN
-- Handle the error here
END;
END;
2. Utilizing RAISE Statements
Another way to handle errors in a PostgreSQL script is to use RAISE statements. These statements allow you to raise custom exceptions when specific conditions are met. For example:
BEGIN
-- Main script logic here
-- Check for a specific condition
IF condition THEN
RAISE EXCEPTION 'Custom error message';
END IF;
END;
3. Logging Errors
In addition to handling errors, it is important to log any errors that occur in a PostgreSQL script. This can help with troubleshooting and debugging issues later on. You can use the RAISE NOTICE statement to log errors to the console or a file. For example:
BEGIN
-- Main script logic here
-- Error handling
BEGIN
-- Specific part of the script where errors may occur
EXCEPTION
WHEN others THEN
RAISE NOTICE 'An error occurred: %', SQLERRM;
END;
END;
4. Conclusion
Implementing error handling in specific parts of a PostgreSQL script is important for improving the reliability and robustness of the script. By using TRY...CATCH blocks, RAISE statements, and logging errors, you can ensure that any issues are caught and handled appropriately. This can help prevent unexpected errors and improve the overall performance of your PostgreSQL scripts.