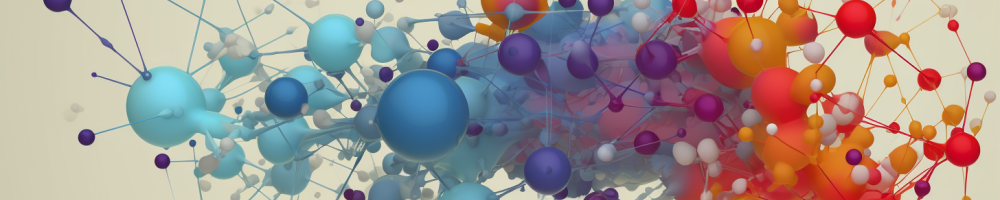
If you are new to React and want to understand Redux and Thunk, you've come to the right place. In this guide, we will explain these concepts using simple terms and examples.
What is Redux?
Redux is a state management library that helps you manage the state of your React application. It follows the Flux architectural pattern and provides a predictable and centralized way to manage data in your app.
With Redux, you can store the data of your application in a single object called the 'store'. The store is immutable, meaning you can't modify it directly. Instead, you dispatch actions to modify the state. Actions are simple JavaScript objects that describe what should happen in your app.
To visualize it, think of the store as a giant container with all your app's data, and actions as small messages that describe what needs to be done with that data. Redux helps you manage this interaction effortlessly.
What is Thunk?
Thunk is a middleware library for Redux. It allows you to write action creators that return functions instead of plain action objects. This is useful when you need to perform async operations inside your action creator, such as making API requests.
Actions returned by thunk-based action creators do not have to be plain objects. They can be functions that receive the 'dispatch' function as an argument. This lets you dispatch multiple actions asynchronously, control the flow of actions, and handle side effects.
Example:
// Redux Actions
const fetchData = () => {
return async (dispatch) => {
dispatch({ type: 'FETCH_START' }); // Dispatching a start action
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
dispatch({ type: 'FETCH_SUCCESS', payload: data }); // Dispatching success action with data
} catch (error) {
dispatch({ type: 'FETCH_ERROR', payload: error.message }); // Dispatching error action with error message
}
};
};
// Redux Reducer
const reducer = (state = initialState, action) => {
switch (action.type) {
case 'FETCH_START':
return { ...state, loading: true };
case 'FETCH_SUCCESS':
return { ...state, loading: false, data: action.payload };
case 'FETCH_ERROR':
return { ...state, loading: false, error: action.payload };
default:
return state;
}
};
In this example, we have an asynchronous action creator called 'fetchData'. Inside this action creator, we dispatch different actions depending on the state of the API request. Using Thunk middleware, we can write the logic to make the API request and handle the response in an async manner.
The reducer handles each dispatched action and updates the state accordingly. In this case, we update the 'loading' state, store the fetched 'data' or display an 'error' message depending on the dispatched action.
Summary
Redux and Thunk are powerful tools for state management and handling asynchronous operations in React. Redux helps you manage the state of your app, while Thunk middleware allows you to write async logic in your action creators.
Understanding and mastering these concepts will enable you to build scalable and efficient React applications.