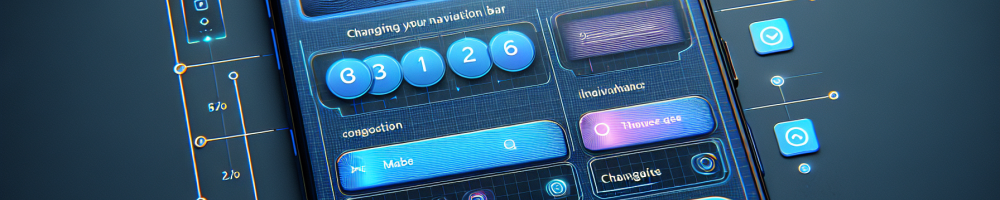
When developing apps with the Expo framework and using navigation with tabs, developers often need the flexibility to update the navigation bar's title based on the active tab or the state of the application. This article outlines the process of dynamically changing the navigation bar title when using Expo and React Navigation with tab navigators.
Setting Up Your Environment
Before you start, make sure you have Expo CLI installed. If not, you can install it using npm:
npm install -g expo-cli
Create a new Expo project by running:
expo init MyTabNavigatorApp
Navigate into your project folder and start the development server:
cd MyTabNavigatorApp
expo start
Installing React Navigation
To handle navigation in the Expo app, install React Navigation and its dependencies:
npm install @react-navigation/native @react-navigation/bottom-tabs react-native-screens react-native-safe-area-context react-native-gesture-handler
Ensure you wrap your app’s root component with the <NavigationContainer>
provided by @react-navigation/native.
Configuring Tab Navigation
Create a bottom tab navigator by importing createBottomTabNavigator
from @react-navigation/bottom-tabs and setting up your tabs:
import { createBottomTabNavigator } from '@react-navigation/bottom-tabs';
const Tab = createBottomTabNavigator();
function MyTabs() {
return (
<Tab.Navigator>
<Tab.Screen name="Home" component={HomeScreen} />
<Tab.Screen name="Settings" component={SettingsScreen} />
</Tab.Navigator>
);
}
Changing the Navigation Bar Title Dynamically
To change the navigation bar title dynamically based on the active tab, use the navigation.setOptions
method within your screen components:
function HomeScreen({ navigation }) {
navigation.setOptions({ title: 'Custom Home Title' });
return <View><Text>Home Screen</Text></View>;
}
function SettingsScreen({ navigation }) {
navigation.setOptions({ title: 'Custom Settings Title' });
return <View><Text>Settings Screen</Text></View>;
}
This approach allows you to customize the navigation bar title based on the specific tab or screen, enhancing the user interface and making your application more intuitive and accessible.