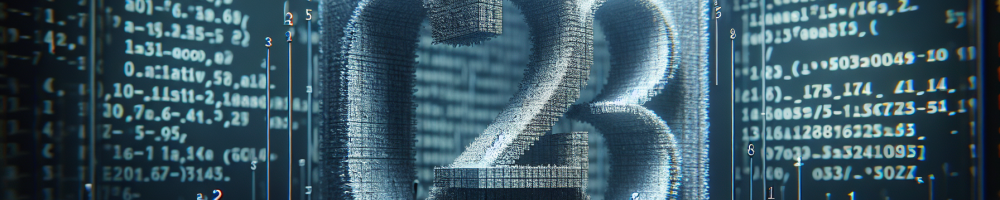
When working with automated tests in Cypress, it might be necessary to verify that a particular element contains only numeric values. This is particularly useful in scenarios where input validation or output display correctness is being tested.
Using Regular Expression Match
To determine if a DOM element's content is strictly numeric, the match()
method combined with a regular expression (regex) can be employed directly in a Cypress test. This approach checks if the text content of an element comprises solely of digits.
Here is a simple example to illustrate:
cy.get('.number-display').invoke('text').then((text) => {
if(text.match(/^\d+$/)) {
console.log('The element contains only numbers.');
} else {
console.log('The element does not contain only numbers.');
}
});
This regular expression /^\d+$/
will match any string that consists only of digits between 0 and 9. Note, however, that this regex does not handle numbers with decimal points. If decimal numbers need to be included, the regex needs to be adjusted accordingly.
Considerations
It's important to consider that the match()
method will return null
if no matches are found, which evaluates as falsy in JavaScript. Ensure appropriate null checks or fallbacks are in place to handle such cases within your Cypress tests.
Add new comment