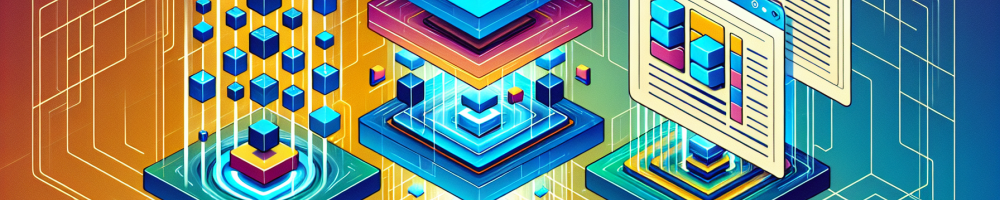
React Native has become a popular framework for developing mobile applications. Underneath its simplicity, it uses Babel to transform JavaScript into a version that’s compatible with older environments. In some cases, developers might need to apply custom Babel transforms to enhance or alter the JavaScript code parsing process for specific needs. Here’s a guide to setting up and running a custom Babel transform in a React Native environment.
Step 1: Install Babel and Required Plugins
Before you can implement custom transformations, you need to ensure that you have Babel installed along with the necessary plugins. In your React Native project, you can install Babel by running:
npm install --save-dev @babel/core
Add any plugins needed for your custom transform, for example:
npm install --save-dev babel-plugin-transform-remove-console
Step 2: Create a Custom Babel Plugin
To create a custom Babel plugin, define a function that exports a function manipulating the Babel types. For example:
module.exports = function(babel) {
const { types: t } = babel;
return {
visitor: {
Identifier(path) {
if (path.node.name === "console") {
path.remove();
}
}
}
};
};
This sample plugin removes all console statements from the JavaScript code.
Step 3: Configure Babel to Use the Custom Plugin
Add your custom plugin configuration in the .babelrc
or babel.config.js
file in your project's root directory:
{
"plugins": ["./path_to_your_custom_plugin"]
}
Replace "./path_to_your_custom_plugin"
with the actual path to your plugin file.
Step 4: Testing Your Babel Transform
After configuring the plugin, run your React Native application to see the effect of the custom Babel transform. If you had a plugin to remove console tags, for instance, none should appear in your final bundle.